TypeORM

TypeORM
is an ORM that can be use on environments such as NodeJS, React Native, and Native Script. It supports both Javascript and Typescript and has an array of different features at your disposal. Sequelize is also an excellent ORM library. I highly recommend you check that one out too.
But, unlike other ORM libraries, TypeORMs
Typescript compatibility is at the forefront of the library, and it shows in features such as decorators. One can declaratively define entities, relations, subscribers, and listeners simplistically.
Code becomes manageable, readable, and easily extensible through the use of decorators. And nothing is better than onboarding on a project and understanding database relationships within a day.
import {
Entity,
PrimaryGeneratedColumn,
Column,
OneToMany
} from "typeorm";
import {Photo} from "./Photo"; @Entity()
export class User {
@PrimaryGeneratedColumn()
id: number; @Column()
name: string; @OneToMany(type => Photo, photo => photo.user)
photos: Photo[];
}
Prompts
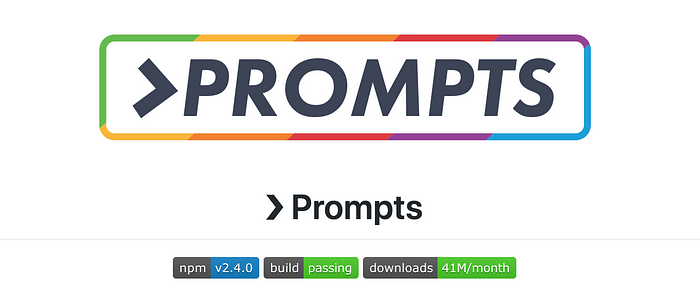
Halfway through the year, I transferred onto a CLI project. It was a fantastic opportunity to see how build-chains such as create-react-app
and create-next-app
are built.
During that project, I was introduced to a really cool command-line user interface npm library called Prompts
. Prompts
is lightweight, supports promises, and provides an array of different ways to prompt an end-user for information. Furthermore, the prompts are beautiful.
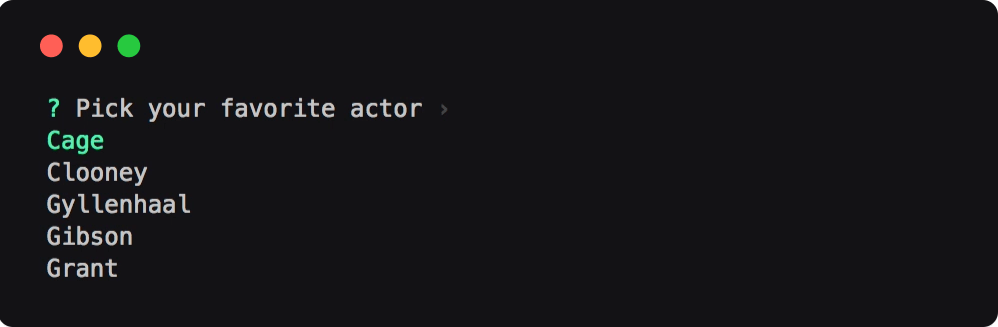
There are many impressive features, such as autocomplete, date picking, multi-select, and my favorite, dynamic prompts.
Sometimes a question to the end-user can yield multiple paths. Traditional conditional structures can grow large and complex. Therefore, they can be difficult to maintain in the long run.
However, Prompts
handles dynamic user input like a dream. It cascades the previous answer, which can then be used to change the follow-up question's nature. Below is a sample of a prompt that verifies a user's age.
const prompts = require('prompts');const questions = [{
type: 'number',
name: 'age',
message: 'How old are you?,
initial: 1,
min: 1,
max: 100
},
{
type: prev => prev < 18 ? 'boolean' : null,
name: 'permissions',
message: 'Has your parent or guardian submitted the Permissions Form?'
},
{
type: (prev, values, prompt) => {
if (prompt.name === 'permissions') {
return prev ? 'boolean' : null
}
return 'boolean'
},
name: 'terms',
message: 'Do you agree to the terms of use?'
}];(async () => {
const response = await prompts(questions);
// if user has agreed to terms of use ... show resource // if not, don't show resource // if user is not the legal age and guardian has not submitted form, show an error message})();
To use the resources beyond the prompts, the end-user must verify their age and accept its terms. If the user is underage (18 years of age) or their guardian has not submitted a form permitting usage, the user cannot proceed and is shown an error message.
I think this library is entertaining! It speaks to my younger self and my initial years learning to program.
Typescript


I am a Typescript believer!
I was pretty reluctant to learn Typescript, fearing it would add unnecessary complexity to already complex code.
Nonetheless, Typescript has provided me with so much more structure and security than VanillaJS. In VanillaJS, there’s often code that is problematic in terms of type security. Sometimes it’s the tiny things developers overlook, such as types, that introduce new bugs and security flaws into a system.
Now I feel confident in the code I write and confident in the code I inherit because I have better insight into how a particular piece of code operates. Typescript makes code easier to read, debug, and maintain.
But, I must admit, there are projects where Typescript does more harm than good, and I found that out the hard way.
Typescript is a superset of Javascript, meaning it’s a layer around JS with more methods, and then it transpiles into Vanilla JS. The compiler and language work together to enforce typing, which then guides you to develop in a particular fashion that’s not supported in VanillaJS.
interface SquareConfig {
color?: string;
width: number;
}function createSquare(config: SquareConfig):
{color: string, area: number} {
return newSquare = {
color: config.color || "white"
area: config.width * config.width
}}
One of my favorite features of Typescript is the use of interfaces. Typescripts core principles revolve around “duck typing”. Duck typing prioritizes verifying the contents of an object or class.
For instance, in the example above, it’s not enough to know that createSquare
accepts an object. Our concerns should be the contents of the object, color
and width
. Creating an interface is a simple and clean way of defining object types without cluttering your function or class definitions.